1. Introduction
The HLI token smart contract is developed on the XRP Ledger (XRPL) blockchain and provides automation of all processes related to the token, including staking, reward distribution, liquidity management and voting through the DAO.
2. Main functions of the smart contract
2.1. Tokenization
Token type: Utility token (HLIT).
Total issue: 100,000,000 HLIT.
Token standard: XRPL (XRP Ledger).
2.2. Token Distribution Schedule
– 50% — Investors
– 10% — Team and Advisors
– 20% — Liquidity Reserve
– 10% — Referral and Marketing Promotions
– 10% — Expense Reserve
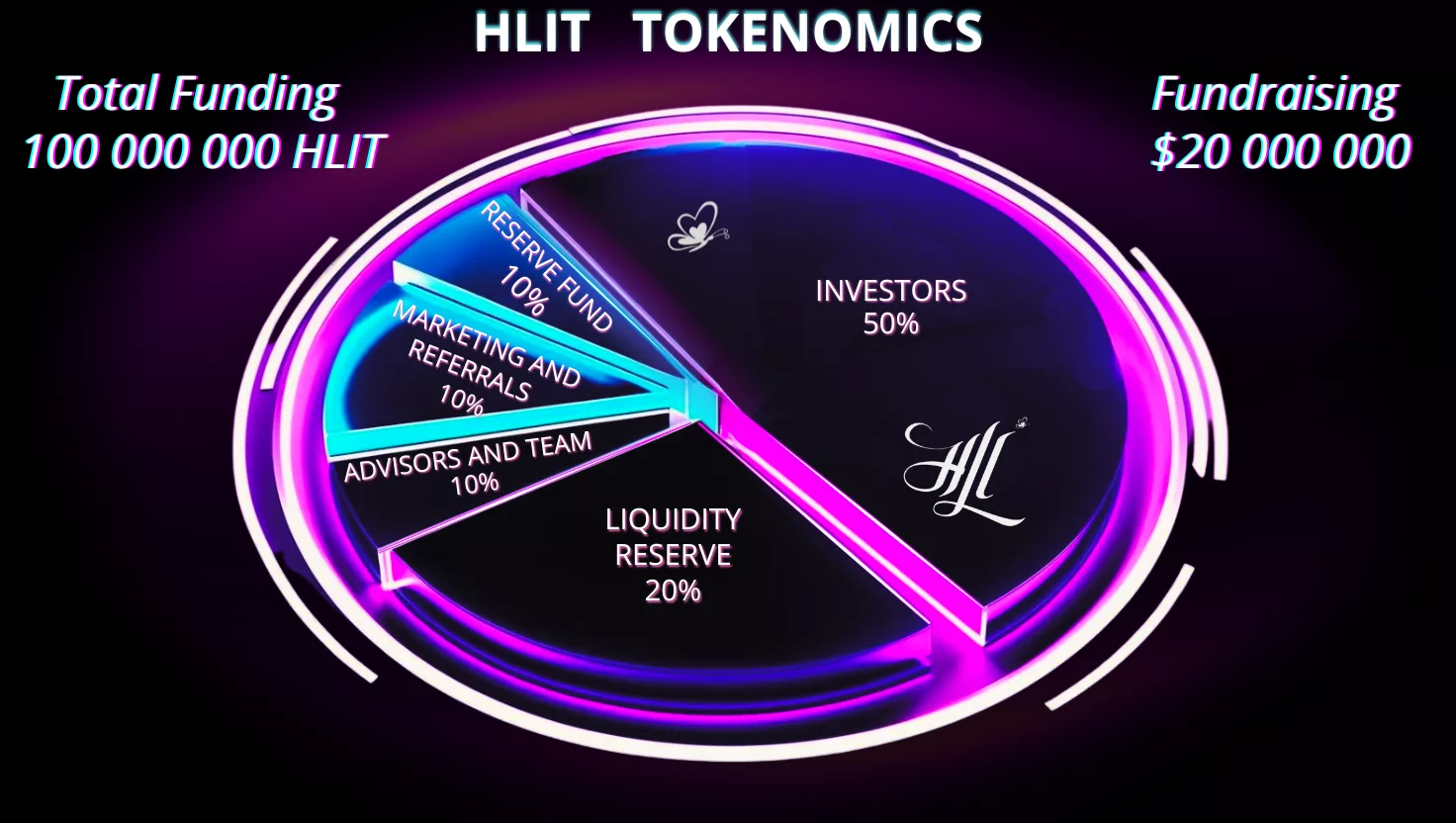
2.3. Token Price Growth Chart
1Q 2025: $0.05 (Private Sale)
2Q 2025: $0.20 (Seed Sale)
3Q 2025: $0.55 (Public Sale 1)
4Q 2025: $1.00 (Public Sale 2)
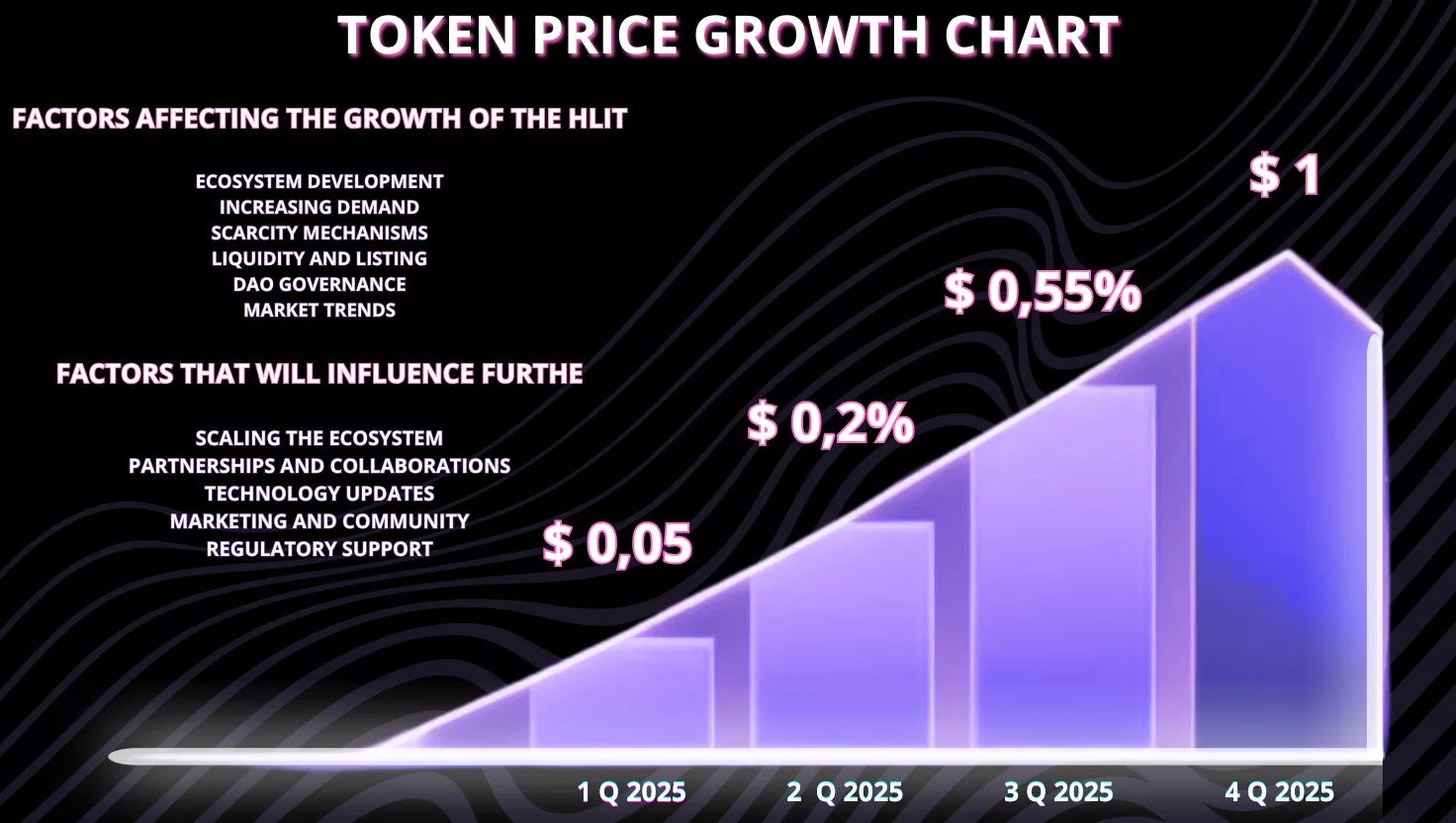
Token code:
const xrpl = require("xrpl");
async function createToken() {
const wallet = xrpl.Wallet.fromSeed('sEd...');// Wallet secret key
const client = new xrpl.Client("wss://s.altnet.rippletest.net:51233");
await client.connect();
const transaction = {
TransactionType: "Payment",
Account: wallet.address,
Destination: "rhBJSzhG8PCWxRSortiqSSo7eTU2DQMpMo",
Amount: {
currency: "HLIT",
issuer: wallet.address,
value: "100000000"
},
};
const prepared = await client.autofill(transaction);
const signed = wallet.sign(prepared);
const result = await client.submitAndWait(signed.tx_blob);
console.log("HLIT token created:", result);
await client.disconnect();
}
createToken().catch(console.error);
3. Reward Mechanics
Staking and Yield:
Investors can freeze tokens and earn income based on the lock-in duration.
Reflection Mechanism:
Investors receive additional tokens from the redistribution of transaction fees.
Yield Farming:
Investors provide liquidity (HLIT/USDT) and earn rewards in HLIT tokens.
Time-Based Rewards:
Investors holding tokens for over 6 months receive additional rewards (airdrop) and access to exclusive offers.
Token Burning:
A portion of tokens is burned to reduce supply and increase the value of remaining tokens.
Referral Program:
Rewards in HLI tokens for bringing new participants.
Community Activity Rewards:
Incentives for sharing information about the token, writing reviews, and generating content
3.1. Automatic Liquidity Staking Program
This method combines staking and automatic liquidity contribution.
- HLIT token holders can lock their tokens on the platform and receive rewards.
- Part of the rewards are automatically directed to the creation of the HLIT/XRP liquidity pool.
- This creates a stable influx of liquidity, reduces volatility and supports price growth.
- Stakers can lock their tokens for periods of: 30, 60, 90, 180 days to receive increased interest rates (APR).
Benefits:
- Passive income: HLI token holders receive rewards for participating in staking.
- Liquidity enhancement: Part of the profit is directed to replenish the liquidity pool.
- Long-term sustainability: The model incentivizes long-term holding of the HLI token.
- Token holding and price volatility reduction: Increased value and demand for the token due to a fixed supply.
Reward Structure:
30 days — 5% per annum.
60 days — 8% per annum.
90 days — 12% per annum.
180 days — 18% per annum.
Loyalty Bonus:
If an investor does not withdraw tokens after the lock-in period, they receive an additional 2% yield.
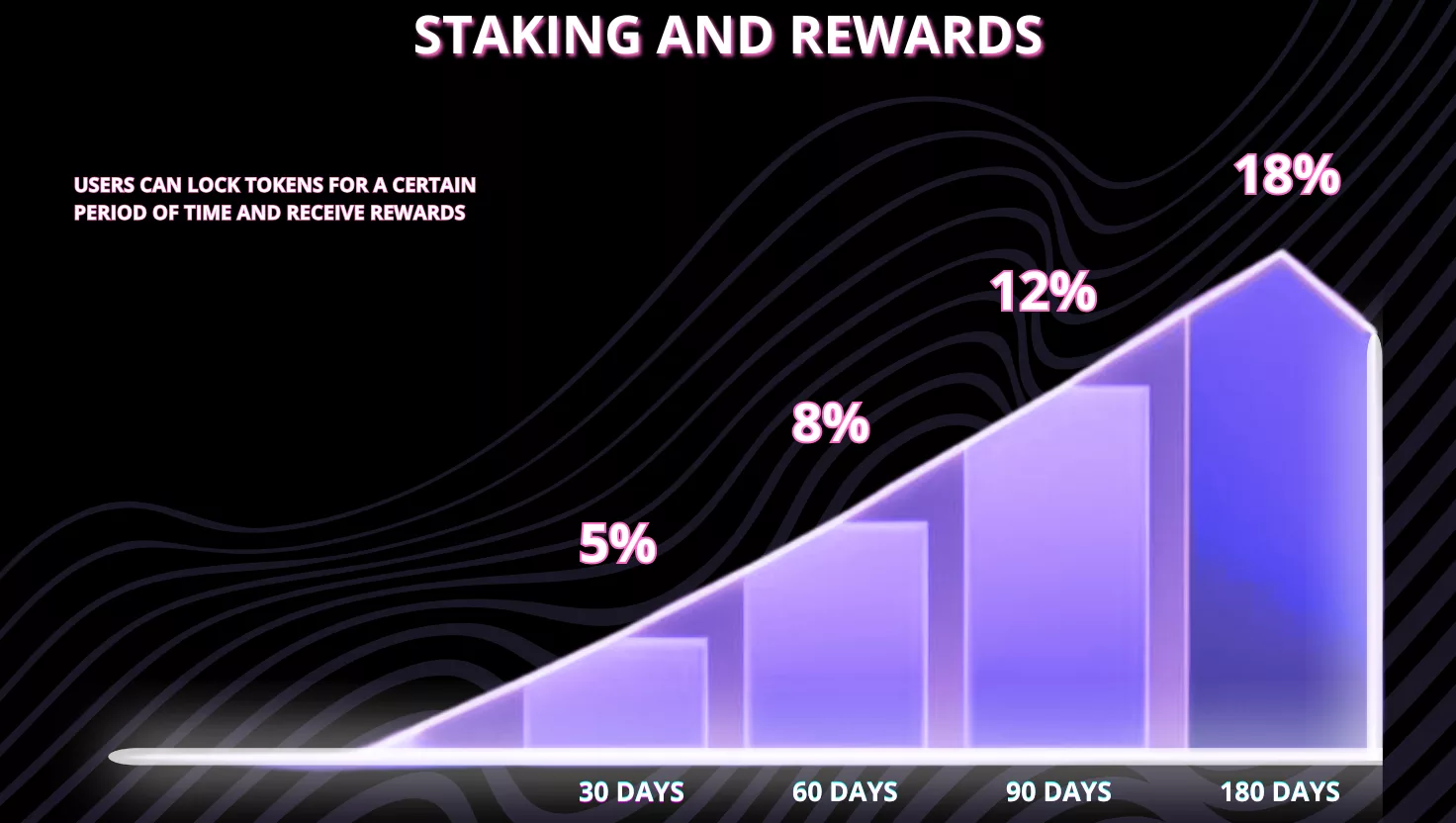
Staking code:
function stakeTokens(uint256 amount, uint256 duration) public {
require(balanceOf[msg.sender] >= amount, "Not enough tokens");
balanceOf[msg.sender] -= amount;
stakedTokens[msg.sender] += amount;
stakingTime[msg.sender] = block.timestamp;
stakingDuration[msg.sender] = duration;
}
function calculateRewards(address user) public view returns (uint256) {
uint256 stakedAmount = stakedTokens[user];
uint256 duration = stakingDuration[user];
uint256 rate;
if (duration == 30 days) rate = 5;
else if (duration == 60 days) rate = 8;
else if (duration == 90 days) rate = 12;
else if (duration == 180 days) rate = 18;
uint256 rewards = (stakedAmount * rate * duration) / (365 * 100);
return rewards;
}
3.2. Reflection Mechanism (Automatic Holder Rewards)
- Each transaction is subject to a 3% fee, of which 1.5% is distributed among all HLIT holders proportionally to the number of their tokens.
- The more tokens an investor holds, the greater their share of the total reward.
- Automatic reward accrual – no need to do anything, just hold the tokens.
Benefits:
- Investors receive “passive income” simply by holding tokens.
- Encourages long-term token holding and reduces selling pressure.
Fee distribution:
- 1.5% is automatically distributed among all token holders.
- 1.5% goes to the liquidity pool or buyback (token burning).
Goal:
Increase token value for holders and generate passive income through ownership.
Reflection Mechanism Code:
function transfer(address to, uint256 value) public returns (bool) {
require(balanceOf[msg.sender] >= value, "Not enough tokens");
uint256 reflectionFee = (value * 15) / 1000; // 1.5%
uint256 liquidityFee = (value * 15) / 1000; // 1.5%
uint256 transferAmount = value - reflectionFee - liquidityFee;
balanceOf[msg.sender] -= value;
balanceOf[to] += transferAmount;
balanceOf[address(this)] += liquidityFee;
// Distribution of reflection
for (address holder : holders) {
balanceOf[holder] += (reflectionFee * balanceOf[holder]) / totalSupply;
}
return true;
}
3.3. Yield Farming
Liquidity Pools: HLIT/USDT
Annual Percentage Yield (APY): HLIT/USDT — 26%
How It Works:
- Investors add liquidity to the pool on DEX and earn 0.5% weekly rewards in HLIT tokens.
- HLIT tokens can be further staked to earn additional income.
Advantages:
- Increases token liquidity on exchanges.
- Investors benefit from trading fees and staking rewards.
Goal:
Maintain exchange liquidity and reduce price volatility.
3.4. Time-Based Rewards
- The longer an investor holds tokens, the greater their reward.
- Holding periods of 6 months and 12 months are tracked through smart contracts.
Benefits:
- Encourages investors to keep tokens in their wallets, reducing sales pressure.
- Helps maintain token price stability.
Rewards:
- After 6 months: A 3% bonus based on the average HLIT balance.
- After 12 months: A 6% bonus based on the average HLIT balance.
- Token airdrops for long-term holders — random distribution of tokens.
Additional Bonuses:
- Access to exclusive NFT airdrops and new tokens from partners.
- Random token distributions for those holding tokens for over 12 months.
- The number and volume of airdrops are determined by the DAO or community voting.
Goal:
To encourage long-term token holding and build a base of loyal holders.
3.5. Buyback and “Burning” of tokens
This method reduces the total supply of tokens, increasing their value to investors.
- A portion of the project’s income or transaction fees is used to purchase tokens on the market.
- The purchased tokens are “burned”, which reduces the total supply and increases the price.
Frequency:
Once a month.
Volume:
1% of the total turnover.
Benefits:
- Increases the value of HLI tokens in the long term.
- Reduces inflation and strengthens investor confidence.
- Automatic buybacks – as soon as liquidity reaches a certain threshold, automatic token purchases are activated.
Goal:
Create a deficit in the market and increase the value of tokens.
3.6. Referral program
- Users receive a referral link to invite new discounts.
- When a new user buys tokens through a referral link, the partner receives a reward in HLIT.
Reward levels:
- Level 1 – 10% of the purchase of referral tokens.
- Level 2 – 5% of the purchase of referral tokens.
- Level 3 – 2% of the purchase of referral tokens.
- Level 4 – 1% of the purchase of referral tokens.
- Level 5 – 0.5% of the purchase of referral tokens.
Additional bonuses:
Top Referral program with bonuses for the best partners.
Benefits:
- Accelerated community growth.
- Reduces marketing and recruitment costs.
Goal:
Increase the number of user bases and increase the popularity of tokens.
3.7. Rewards for community activity
- Investors complete tasks (posts on social networks, writing articles) and receive tokens.
- Tasks are checked manually or using smart contracts.
Benefits:
- Increases brand awareness.
- Turns investors into project ambassadors.
Examples of rewards:
- Rewards for reposts and comments on social networks.
- Bonus for creating content (reviews, videos, articles)
4. HLI Token Liquidity Pools
Creating liquidity for the HLI token is a crucial aspect of its market stability.
Liquidity ensures quick token purchases and sales without significant price fluctuations.
Token HLI Design:
Built as a multifunctional token on the XRP Ledger (XRPL) platform. To ensure stability, accessibility, and long term growth, a comprehensive liquidity pool strategy (LP) is proposed.
Goals of Liquidity Pools:
- Market Liquidity: Simplify HLIT trading on decentralized (DEX) and centralized exchanges (CEX).
- Price Volatility Reduction: Prevent price manipulation through sufficient liquidity.
- DAO Governance: Allow community participation in liquidity management.
- Staking Rewards: Provide rewards to users contributing liquidity.
- Token Utility: Integrate staking, rewards, and voting rights into liquidity activities.
4.1. Liquidity Pools on Decentralized Exchanges (DEX)
- Targeted DEX: Sologenic DEX or GateHub (support XRP Ledger tokens).
- Liquidity Pairs: HLIT/USDT.
- Liquidity Sources: Initial liquidity provided by the project’s reserve fund.
- Community contributions: DAO members and the community can contribute liquidity and earn pool commission rewards.
Smart Contract Logic for LP:
Users deposit HLIT and USDT in a 50/50 ratio.
In return, they receive LP tokens representing their share of the pool.
LP tokens can be staked to earn rewards from every transaction in the pool.
Fee Structure:
Liquidity providers receive a percentage of trading fees.
1.5% of each transaction is distributed among LP token holders proportionally.
DAO can vote to change the fee structure.
4.2. Liquidity Pools on Centralized Exchanges (CEX)
Liquidity Source:
Provided by the HLI project and early investors.
Market Making: The exchange may assist in maintaining price stability.
Target CEX Platforms:
KuCoin, or Bybit
Liquidity Strategy:
- Listing HLIT on CEX to expand market reach.
- Providing liquidity via market-making using treasury funds.
- Agreements with CEX platforms to incentivize liquidity and offer bonuses to early participants.
Benefits:
- Access to a Broad Audience: Enables users unfamiliar with DEX to purchase tokens on centralized platforms.
- Scalability: Connecting to major exchanges facilitates entry into international markets.
- Market Depth: Centralized exchanges provide higher liquidity.
4.3. Automation through DAO and Managed Liquidity Pool
The creation of a DAO-managed liquidity pool is an innovative approach that involves managing liquidity through decentralized voting by token holders.
- HLIT holders participate in DAO governance. Investor tokens serve as a “vote” in the DAO.
- The voting process is managed by smart contracts on the XRP Ledger.
- The number of votes depends on the number of tokens a user holds in their balance.
- The liquidity pool can be funded through DAO fees or the project’s treasury.
- DAO participants earn profits from liquidity fees and bonuses for active voting.
Advantages:
- Transparency: All liquidity is controlled by the DAO, enhancing community trust.
- Token Holder Rewards: HLIT holders can vote for LP rewards and increased yields. Transaction fees are directed to the DAO and redistributed among token holders.
- Flexibility: Pool conditions can be adjusted based on DAO voting.
- Increased Investor Engagement: Participants feel more valued in the project and are more inclined to hold onto their tokens.
Voting System:
- Proposal Submission: Any DAO member can submit a proposal.
- Voting Duration: 72 hours.
- Quorum: 20% of DAO token holders must participate for the vote to be valid.
Code for DAO:
struct Proposal {
string description;
uint256 voteCount;
bool executed;
}
mapping(uint256 => Proposal) public proposals;
uint256 public proposalCount;
function createProposal(string memory description) public {
proposals[proposalCount] = Proposal({
description: description,
voteCount: 0,
executed: false
});
proposalCount++;
}
function vote(uint256 proposalId, uint256 amount) public {
require(balanceOf[msg.sender] >= amount, "Not enough tokens");
proposals[proposalId].voteCount += amount;
}
Smart contract operation scheme
- The user locks tokens for staking.
- The smart contract automatically distributes rewards.
- The DAO manages liquidity and makes decisions.
- The reflection mechanism distributes fees between holders.
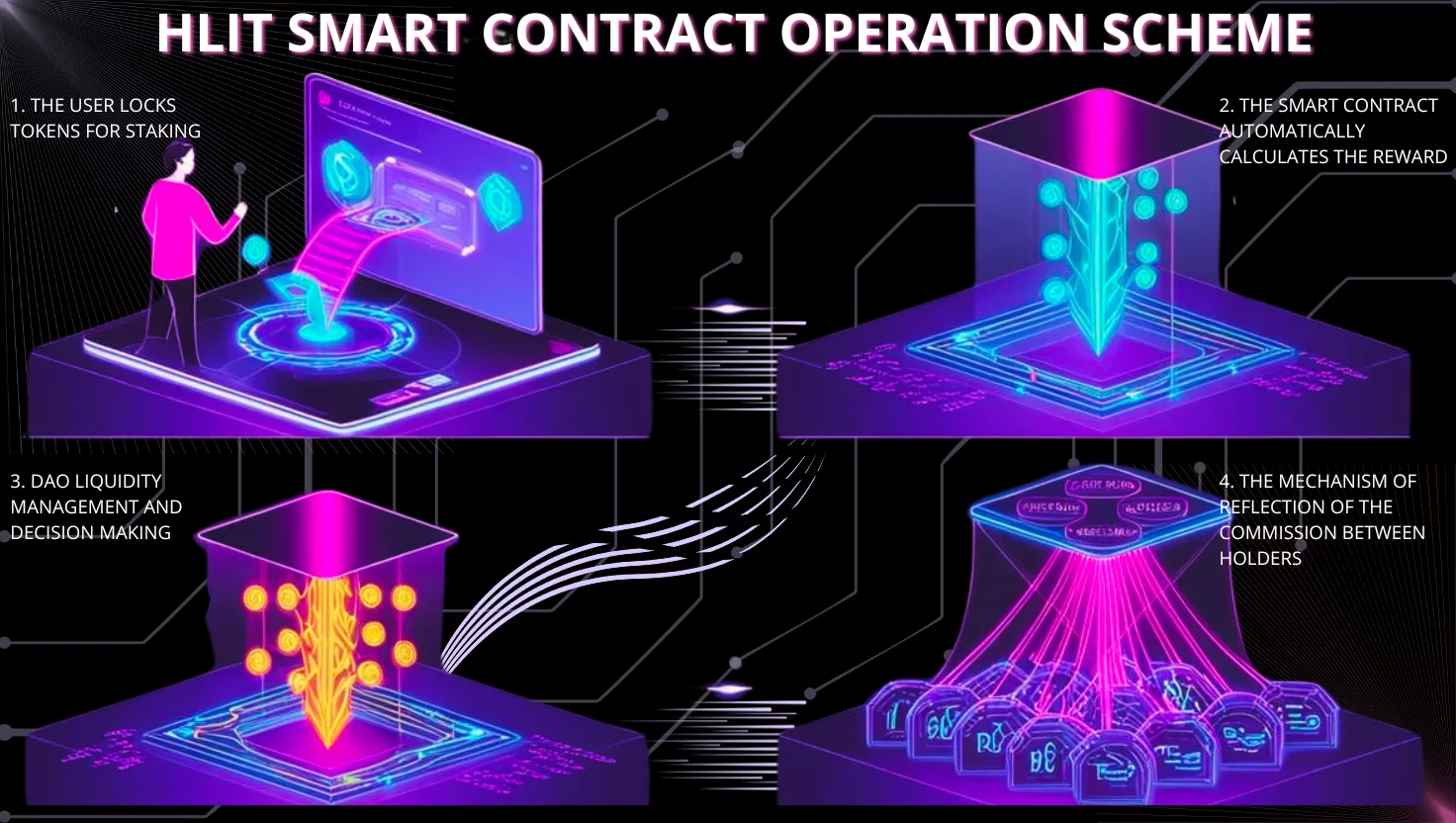
4.4. Custom Liquidity Pool with Bot Protection or Vesting
To protect against bots and dishonest traders, a liquidity pool with automatic protection is created. This prevents automated trading scripts that can deplete liquidity or manipulate prices.
Protection Mechanisms:
- The HLI token is programmed with anti-bot protection (analyzing transaction frequency, introducing a delay
- between purchase and sale).
- Limits are set on token purchases and sales on both DEX and CEX platforms.
- Smart liquidity control — automatic blocking of large transactions.
- Implementation of KYC/AML mechanisms to exclude multi-account setups.
- Automated monitoring of suspicious transactions.
Dynamic Fees:
- Temporary fee increases for rapid transactions to deter bots.
- Funds collected from bots are redirected to the DAO treasury.
Advantages:
- Bot Protection: Automatic blocking of overly frequent transactions or unusual activity.
- Price Control: Reduced risk of “pump-and-dump” attacks.
- Liquidity Stability: Protects liquidity from sharp price manipulation.
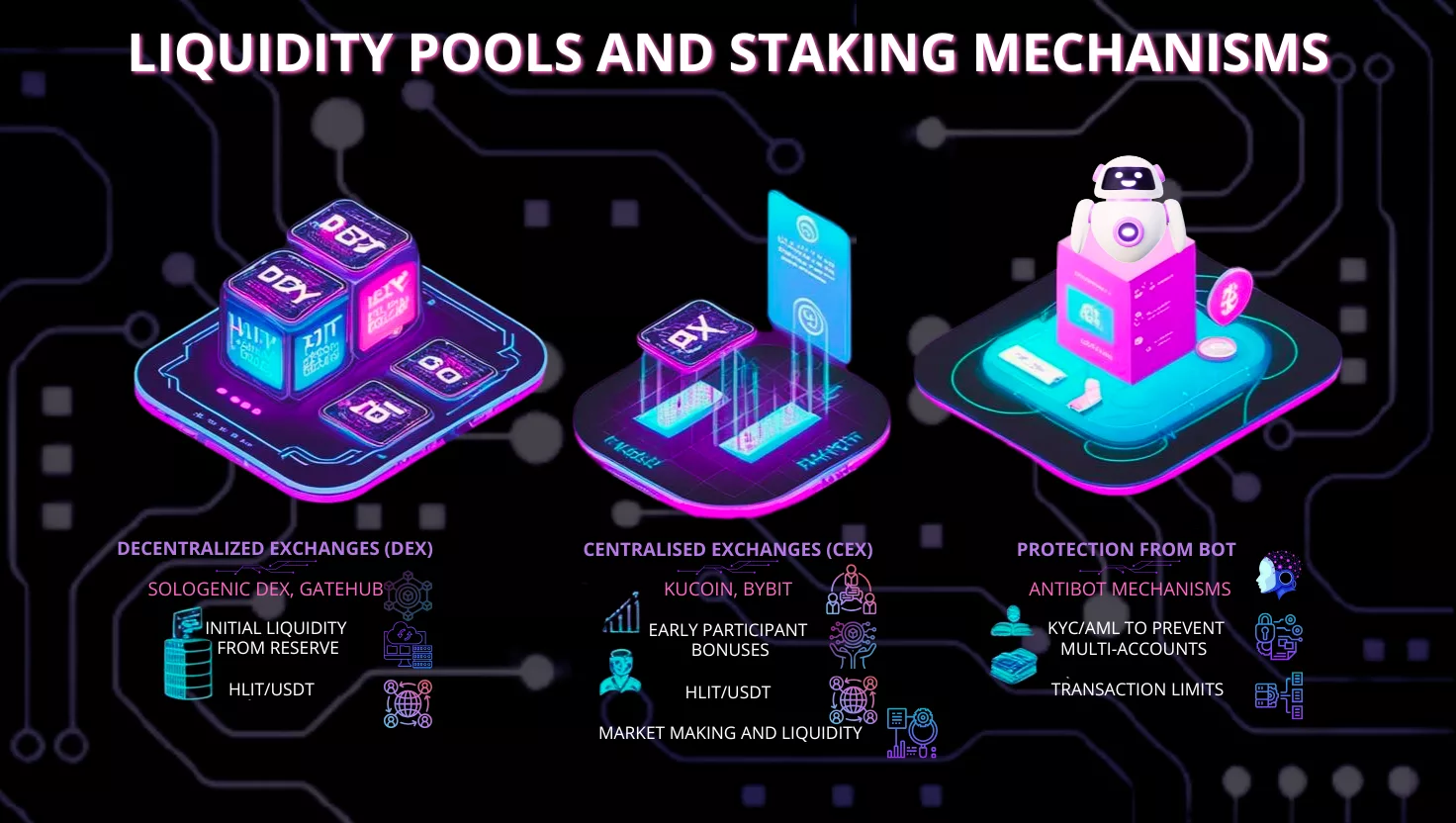
5. Conclusion
The HLI token smart contract ensures full transparency, automation, and security of all processes. Investors can participate in staking, receive passive income, and participate in project management through DAO.
6. Additional Resources
- Whitepaper:
- GitHub: underway.
- Technical documentation: underway.
*Note to Readers:
The code snippets provided in this smart contract documentation are illustrative examples intended to demonstrate the functionality and logic of the HLI token ecosystem. These examples are designed to help you understand the core mechanisms, such as staking, rewards distribution, reflection, and DAO governance.
Please note that the final, production-ready code will be implemented and thoroughly audited by certified blockchain security firms before deployment. The audit process ensures that the smart contract is secure, efficient, and free from vulnerabilities. Once the audit is completed, the verified code will be made publicly available for transparency and trust.
We are committed to delivering a robust and secure smart contract that aligns with the highest industry standards.
Thank you for your understanding and trust in the HLI project.